Overview
Every Drupal site builder works with blocks. Blocks are an essential building component in Drupal. Block configuration allows for the site builder to determine various conditions in which the block should be shown. Some examples are to show the block only for a given content type, a given role, or a given path.
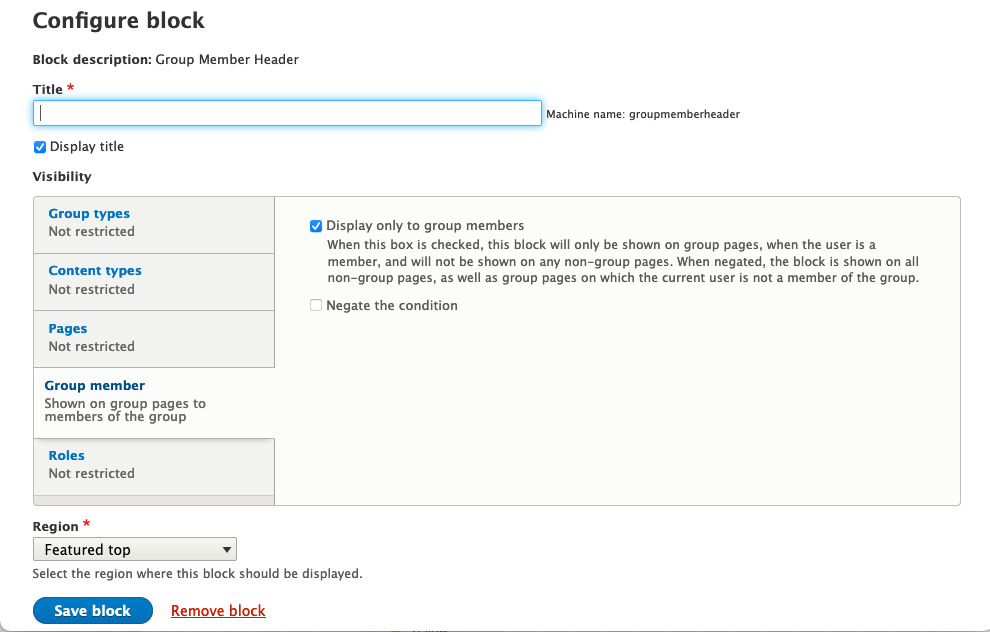
Behind the scenes, Drupal uses the Condition Plugin API to define conditions under which blocks are shown. This API allows developers to create Drupal plugins that act as new conditions that can be selected when placing blocks, allowing developers to create dynamic custom conditions that meet specific and complex requirements on websites.
This tutorial will outline the process of creating a new Condition Plugin API plugin, extending the types of conditions that can be applied to block configuration. This tutorial will use the Groups module. A new condition will be created whereby the block is only shown if the user is a member of the current group.
Requirements
This tutorial requires an understanding of how to create a Drupal module, what a Drupal plugin is, how to register a plugin through class annotation, and how to inject services as dependencies in Drupal OOP. These are core concepts for Drupal development, so if you don't yet understand them and intend to develop in Drupal, it is worth taking the time to read and have somewhat of an understanding before reading this tutorial.The tutorial it is dependent upon the Groups module, without which the code shown will throw errors and not work.
Goal
The goal is to create a new tab in the vertical tabs on the block configuration page. The tab will add a checkbox for user is member of current group. As conditions come with a negate condition checkbox, there will be three possible states for this condition:
- Neither box checked - this is the default state. This condition does nothing, and the block will be shown on all pages.
- The display to group members box is checked, and the negate the condition box is unchecked. The block is shown only on group pages, if the current user is a member of the group.
- Both boxes are checked. The block is shown on non-group pages, and on group pages where the current user is not a member of the group.
Note that when the display to group members box is unchecked, the negate the condition checkbox has no effect. This example will therefore set this checkbox to only display when the display to group members box has been checked.
A Note on Negating Conditions
When the negate this condition box is checked, the condition is evaluated, and if it is FALSE
, the negated condition is satisfied. In the case of this tutorial, the condition evaluates to TRUE
when the user is currently in a group context AND they are a member of the group. When the negate checkbox is checked, if the user is not on a group page, the condition evaluates to FALSE
, so the negated condition is satisfied, and the block is shown. If the user is on a group page, but is not a member, the condition evaluates to FALSE
, meaning the negated condition is satisfied and the block is shown. If the user is on a group page, and is a member, the condition evaluates to TRUE
, so the negated condition is not satisfied and the block is not shown.
Process
The following steps are required:
- Extend
ConditionPluginBase
to declare a new@Condition
plugin. - Register the default configuration for the condition.
- Create the configuration form to be shown on the block configuration page.
- Create a summary.
- Implement the
evalute()
method to determine if the condition has been met. - Add a cache context for members of the current group, so that the condition is re-evaluated for members and non-members.
- (Optional) Set the negate checkbox to only show when the condition is enabled.
- (Optional) Add custom JavaScript to show the summary in the vertical tabs.
Step 1: Declare a new Condition Plugin
Creating a new vertical tab on the block configuration page requires creating a new Condition Plugin API plugin. After clearing the registry, Drupal will automatically add it to the vertical tabs on the block configuration page.
@Condition
plugins extend ConditionPluginBase, which implements ConditionInterface. @Condition
Plugins go into the Drupal\[module]\Plugin\Condition
namespace. The class annotation defines a new @Condition
plugin.
/** * @Condition( * id = "group_member", * label = @Translation("Group member"), * ) */
The example uses the current_route_match_service, injected as $this->currentRouteMatch
, and the current_user service, injected as $this->currentUser
.
<?php namespace Drupal\[MODULE]\Plugin\Condition; use Drupal\Core\Condition\ConditionPluginBase; use Drupal\Core\Form\FormStateInterface; use Drupal\Core\Plugin\ContainerFactoryPluginInterface; use Drupal\Core\Routing\ResettableStackedRouteMatchInterface; use Drupal\Core\Session\AccountProxyInterface; use Symfony\Component\DependencyInjection\ContainerInterface; /** * Provides a condition for members of the current group. * * This condition evaluates to TRUE when in a group context, and the current * user is a member of the group. When the condition is negated, the condition * is shown when either not in group context, or in group context but the * current user is not a member of the group. * * @Condition( * id = "group_member", * label = @Translation("Group member"), * ) */ class GroupMember extends ConditionPluginBase implements ContainerFactoryPluginInterface { /** * The current route match service. * * @var \Drupal\Core\Routing\ResettableStackedRouteMatchInterface */ protected $currentRouteMatch; /** * The current user. * * @var \Drupal\Core\Session\AccountProxyInterface */ protected $currentUser; /** * Creates a new PodcastType instance. * * @param array $configuration * The plugin configuration. * @param string $plugin_id * The plugin_id for the plugin instance. * @param mixed $plugin_definition * The plugin implementation definition. * @param \Drupal\Core\Routing\ResettableStackedRouteMatchInterface $currentRouteMatch * The curretn route match service. * @param \Drupal\Core\Session\AccountProxyInterface $currentUser * The current user. */ public function __construct( $plugin_id, $plugin_definition, ResettableStackedRouteMatchInterface $currentRouteMatch, AccountProxyInterface $currentUser ) { parent::__construct($configuration, $plugin_id, $plugin_definition); $this->currentRouteMatch = $currentRouteMatch; $this->currentUser = $currentUser; } /** * {@inheritdoc} */ public static function create(ContainerInterface $container, array $configuration, $plugin_id, $plugin_definition) { return new static( $configuration, $plugin_id, $plugin_definition, $container->get('current_route_match'), $container->get('current_user') ); } }
Step 2: Register the Default Configuration
The default configuration needs to be declared for the condition. This condition will be disabled by default, as blocks should be shown for all users on all pages by default, and only have limitations placed on them when the condition is enabled.
/** * {@inheritdoc} */ public function defaultConfiguration() { // The default configuration will be have the block hidden (0). return ['show' => 0] + parent::defaultConfiguration(); }
Step 3: Create the Configuration Form
First, the Next, the configuration form is defined with a checkbox to enable the condition.
/** * {@inheritdoc} */ // Define the checkbox to enable the condition. $form['show'] = [ '#title' => $this->t('Display only to group members'), '#type' => 'checkbox', // Use whatever value is stored in cofiguration as the default. '#default_value' => $this->configuration['show'], '#description' => $this->t('When this box is checked, this block will only be shown on group pages, when the user is a member, and will not be shown on any non-group pages. When negated, the block is shown on all non-group pages, as well as group pages on which the current user is not a member of the group.'), ]; return parent::buildConfigurationForm($form, $form_state); }
Finally, a submit handler is created to save the submitted value to configuration.
/** * {@inheritdoc} */ // Save the submitted value to configuration. $this->configuration['show'] = $form_state->getValue('show'); parent::submitConfigurationForm($form, $form_state); }
Step 4: Create a Summary
ConditionBase implements ConditionInterface, which requires the method summary(). This method outputs a human-readable description of the condition settings. The summary method returns a different value depending on the which of three configuration states is set:
- Condition is not enabled
- Condition is enabled
- Condition is enabled and negated
/** * {@inheritdoc} */ public function summary() { if ($this->configuration['show']) { // Check if the 'negate condition' checkbox was checked. if ($this->isNegated()) { // The condition is enabled and negated. return $this->t('Shown on non-group pages and on group pages to non-members'); } else { // The condition is enabled. return $this->t('Shown on group pages to members of the group'); } } // The condition is not enabled. return $this->t('Not Restricted'); }
Unfortunately, the summary is not shown by default in the vertical tabs on the block configuration page. The summary created above allows developers to use conditions programmatically and output human readable summaries that can be used in logs, or messages, or even dashboards.
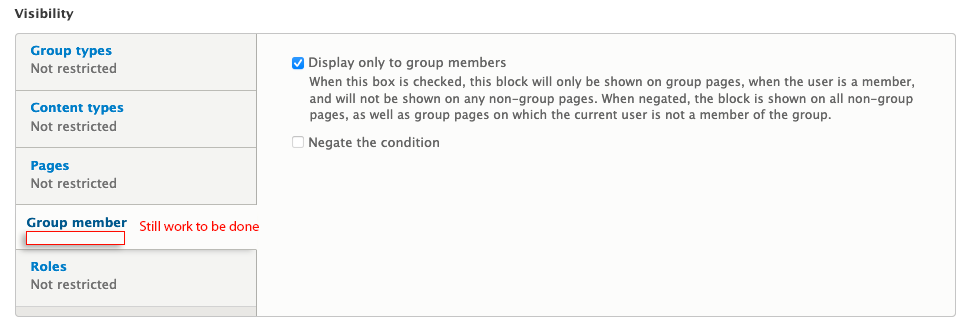
Step 8 will show how to add a summary on the vertical tabs.
Step 5: Evaluate the Condition
ConditionBase implements ConditionInterface, which requires the method evaluate(). This method returns a boolean indicating whether or not the current condition has been met.
/** * {@inheritdoc} */ public function evaluate() { // Determine if in a group context. if ($group = $this->currentRouteMatch->getParameter('group')) { // Return a boolean based on whether the user is a member of the group. return (bool) $group->getMember($this->currentUser); } // Not in a group context. return FALSE; }
Step 6: Add a Cache Context
As it stands right now, the condition will work correctly the first time the condition is evaluated, and the result is cached. So, if the condition is enabled, and the first user to visit a group page is a member of the group, the condition evaluates to TRUE
and the block is rendered. The problem is when a non-member visits the group, the condition has been cached as TRUE
, so the user sees the block, even though they are not a member of the group.
The solution to this is to add cache contexts. The cache context tells Drupal that the condition should be re-evaluated in various contexts. For the example in this tutorial, the condition will have a different result for members and non-members of the group, therefore a cache context must be created that tells the system to cache a different result for each of these contexts.
Cache contexts can be added by extending ContextAwarePluginTrait::getCacheContexts(), and adding a cache context for user.is_group_member:%group_id
.
/** * {@inheritdoc} */ public function getCacheContexts() { // Get the default cache contexts. $contexts = parent::getCacheContexts(); // Check if in group context. if ($group = $this->currentRouteMatch->getParameter('group')) { // Add a context for members of the current group, so that the block // differs between members and non-members. $contexts[] = 'user.is_group_member:' . $group->id(); } return $contexts; }
Step 7 (Optional): Hide the Negate checkbox when the condition is disabled
The negate the condition checkbox only has meaning when the condition is enabled, as the evaluate()
method is not called when the condition is not enabled. As such, it does not make sense to show the negate checkbox when the condition is not enabled. This checkbox is provided automatically by the API, however it is always shown regardless of whether the condition is enabled. To improve the UX, the negate checkbox can be set to be hidden until the display only to group members checkbox has been clicked.
This is done by implementing hook_form_FORM_ID_alter() as hook_form_block_form_alter()
in the [MODULE].module
file for the module, and adding #states
to the negate checkbox.
/** * Implements hook_form_FORM_ID_alter(). */ function HOOK_form_block_form_alter(&$form, FormStateInterface $form_state) { // Add a #state for visibility tot he group member negate checkbox. $form['visibility']['group_member']['negate']['#states'] = [ 'visible' => [ '[data-drupal-selector="edit-visibility-group-member-show"]' => ['checked' => TRUE], ], ]; } }
Step 8 (Optional): Add JavaScript for the Settings Summary
Drupal core adds settings summary to the vertical tabs on the block settings page for tabs provided by core. Custom modules must add their own JavaScript and tie into the API to show the summary.
The first step is to create a library in [MODULE].libraries.yml
linking to the .js file.
block: js: js/group_member_condition.js: {} dependencies: - 'block/drupal.block'
Then, back to hook_form_block_form_alter()
(see step 5) to attach the library to the form.
/** * Implements hook_form_FORM_ID_alter(). */ function HOOK_form_block_form_alter(&$form, FormStateInterface $form_state) { // Attach the library for the vertical tab. $form['visibility_tabs']['#attached']['library'][] = '[MODULE]/block'; // Add a #state for visibility tot he group member negate checkbox. $form['visibility']['group_member']['negate']['#states'] = [ 'visible' => [ '[data-drupal-selector="edit-visibility-group-member-show"]' => ['checked' => TRUE], ], ]; } }
You will need to replace [MODULE]
with your module key.
And finally, group_member_condition.js. Note that for consistency's sake, the text for the summary messages should be manually aligned with the text from the summary()
method of the plugin (see step 3).
/** * @file * Sets up the summary for group members on vertical tabs of block forms. */ (function ($, Drupal) { 'use strict'; function checkboxesSummary(context) { // Determine if the condition has been enabled (the box is checked). var conditionChecked = $(context).find('[data-drupal-selector="edit-visibility-group-member-show"]:checked').length; // Determine if the negate condition has been enabled (the box is checked). var negateChecked = $(context).find('[data-drupal-selector="edit-visibility-group-member-negate"]:checked').length; if (conditionChecked) { if (negateChecked) { // Both boxes have been checked. return Drupal.t("Shown on non-group pages and on group pages to non-members"); } // The condition has been enabled. return Drupal.t("Shown on group pages to members of the group"); } // The condition has not been enabled and is not negated. return Drupal.t('Not restricted'); } /** * Provide the summary information for the block settings vertical tabs. * * @type {Drupal~behavior} * * @prop {Drupal~behaviorAttach} attach * Attaches the behavior for the block settings summaries. */ Drupal.behaviors.blockSettingsSummaryGroupMember = { attach: function () { // Only do something if the function drupalSetSummary is defined. if (jQuery.fn.drupalSetSummary !== undefined) { // Set the summary on the vertical tab. $('[data-drupal-selector="edit-visibility-group-member"]').drupalSetSummary(checkboxesSummary); } } }; }(jQuery, Drupal));
Using Conditions Programmatically
Conditions can also be used programatically in other ways on Drupal sites. Here is an example how the condition created above could be used in a programmatic context to determine if the current user is a member of the current group:
$condition = \Drupal::service('plugin.manager.condition')->createInstance('group_member'); $condition->setConfiguration(['show' => 1]); // Test if the condition is satisfied - IE the user is in a group context, and // is a member of the group. if ($condition->evaluate()) { \Drupal::messenger()->addStatus(t('The condition was evaluated as %message', [ '%message' => $condition->summary(), ]); }
Summary
This tutorial examined the Condition Plugin API for Drupal, providing an example of creating a new condition to restrict block visibility to group members with the Groups module. After reading this tutorial you should have an understanding of how this API works, and how to create your own custom conditions for blocks on your Drupal sites.
Complete Code
GroupMember.php:
<?php namespace Drupal\[MODULE]\Plugin\Condition; use Drupal\Core\Condition\ConditionPluginBase; use Drupal\Core\Form\FormStateInterface; use Drupal\Core\Plugin\ContainerFactoryPluginInterface; use Drupal\Core\Routing\ResettableStackedRouteMatchInterface; use Drupal\Core\Session\AccountProxyInterface; use Symfony\Component\DependencyInjection\ContainerInterface; /** * Provides a condition for members of the current group. * * This condition evaluates to TRUE when in a group context, and the current * user is a member of the group. When the condition is negated, the condition * is shown when either not in group context, or in group context but the * current user is not a member of the group. * * @Condition( * id = "group_member", * label = @Translation("Group member"), * ) */ class GroupMember extends ConditionPluginBase implements ContainerFactoryPluginInterface { /** * The current route match service. * * @var \Drupal\Core\Routing\ResettableStackedRouteMatchInterface */ protected $currentRouteMatch; /** * The current user. * * @var \Drupal\Core\Session\AccountProxyInterface */ protected $currentUser; /** * Creates a new PodcastType instance. * * @param array $configuration * The plugin configuration. * @param string $plugin_id * The plugin_id for the plugin instance. * @param mixed $plugin_definition * The plugin implementation definition. * @param \Drupal\Core\Routing\ResettableStackedRouteMatchInterface $currentRouteMatch * The curretn route match service. * @param \Drupal\Core\Session\AccountProxyInterface $currentUser * The current user. */ public function __construct( $plugin_id, $plugin_definition, ResettableStackedRouteMatchInterface $currentRouteMatch, AccountProxyInterface $currentUser ) { parent::__construct($configuration, $plugin_id, $plugin_definition); $this->currentRouteMatch = $currentRouteMatch; $this->currentUser = $currentUser; } /** * {@inheritdoc} */ public static function create(ContainerInterface $container, array $configuration, $plugin_id, $plugin_definition) { return new static( $configuration, $plugin_id, $plugin_definition, $container->get('current_route_match'), $container->get('current_user') ); } /** * {@inheritdoc} */ public function defaultConfiguration() { // Set the default value for the checkbox on the block configuration page. // This condition is not be enabled by default, so the default is set to // zero. return ['show' => 0] + parent::defaultConfiguration(); } /** * {@inheritdoc} */ // Define the checkbox to enable the condition. $form['show'] = [ '#title' => $this->t('Display only to group members'), '#type' => 'checkbox', // Use whatever value is stored in cofiguration as the default. '#default_value' => $this->configuration['show'], '#description' => $this->t('When this box is checked, this block will only be shown on group pages, when the user is a member, and will not be shown on any non-group pages. When negated, the block is shown on all non-group pages, as well as group pages on which the current user is not a member of the group.'), ]; return parent::buildConfigurationForm($form, $form_state); } /** * {@inheritdoc} */ // Save the submitted value to configuration. $this->configuration['show'] = $form_state->getValue('show'); parent::submitConfigurationForm($form, $form_state); } /** * {@inheritdoc} */ public function summary() { if ($this->configuration['show']) { // Check if the 'negate condition' checkbox was checked. if ($this->isNegated()) { // The condition is enabled and negated. return $this->t('Shown on non-group pages and on group pages to non-members'); } else { // The condition is enabled. return $this->t('Shown on group pages to members of the group'); } } // The condition is not enabled. return $this->t('Not Restricted'); } /** * {@inheritdoc} */ public function evaluate() { // Determine if in a group context. if ($group = $this->currentRouteMatch->getParameter('group')) { // Return a boolean based on whether the user is a member of the group. return (bool) $group->getMember($this->currentUser); } // Not in a group context. return FALSE; } /** * {@inheritdoc} */ public function getCacheContexts() { $contexts = parent::getCacheContexts(); // Check if in group context. if ($group = $this->currentRouteMatch->getParameter('group')) { // Add a context for members of the current group, so that the block // differs between members and non-members. $contexts[] = 'user.is_group_member:' . $group->id(); } return $contexts; } }
[MODULE].module:
/** * Implements hook_form_FORM_ID_alter(). */ function HOOK_form_block_form_alter(&$form, FormStateInterface $form_state) { $form['visibility_tabs']['#attached']['library'][] = '[MODULE]/block'; // Add a #state for visibility tot he group member negate checkbox. $form['visibility']['group_member']['negate']['#states'] = [ 'visible' => [ '[data-drupal-selector="edit-visibility-group-member-show"]' => ['checked' => TRUE], ], ]; } }
[MODULE].libraries.yml:
block: version: 'VERSION' js: js/group_member_condition.js: {} dependencies: - 'block/drupal.block'
group_member_condition.js:
/** * @file * Sets up the summary on vertical tabs of block forms. */ /*global jQuery, Drupal*/ (function ($, Drupal) { 'use strict'; /** * Create a summary for checkboxes in the provided context. * * @param {HTMLDocument|HTMLElement} context * A context where one would find checkboxes to summarize. * * @return {string} * A string with the summary. */ function checkboxesSummary(context) { // Determine if the condition has been enabled (the box is checked). var conditionChecked = $(context).find('[data-drupal-selector="edit-visibility-group-member-show"]:checked').length; // Determine if the negate condition has been enabled (the box is checked). var negateChecked = $(context).find('[data-drupal-selector="edit-visibility-group-member-negate"]:checked').length; if (conditionChecked) { if (negateChecked) { // Both boxes have been checked. return Drupal.t("Shown on non-group pages and on group pages to non-members"); } // The condition has been enabled. return Drupal.t("Shown on group pages to members of the group"); } // The condition has not been enabled and is not negated. return Drupal.t('Not restricted'); } /** * Provide the summary information for the block settings vertical tabs. * * @type {Drupal~behavior} * * @prop {Drupal~behaviorAttach} attach * Attaches the behavior for the block settings summaries. */ Drupal.behaviors.blockSettingsSummaryGroupMember = { attach: function () { // Only do something if the function drupalSetSummary is defined. if (jQuery.fn.drupalSetSummary !== undefined) { // Set the summary on the vertical tab. $('[data-drupal-selector="edit-visibility-group-member"]').drupalSetSummary(checkboxesSummary); } } }; }(jQuery, Drupal));